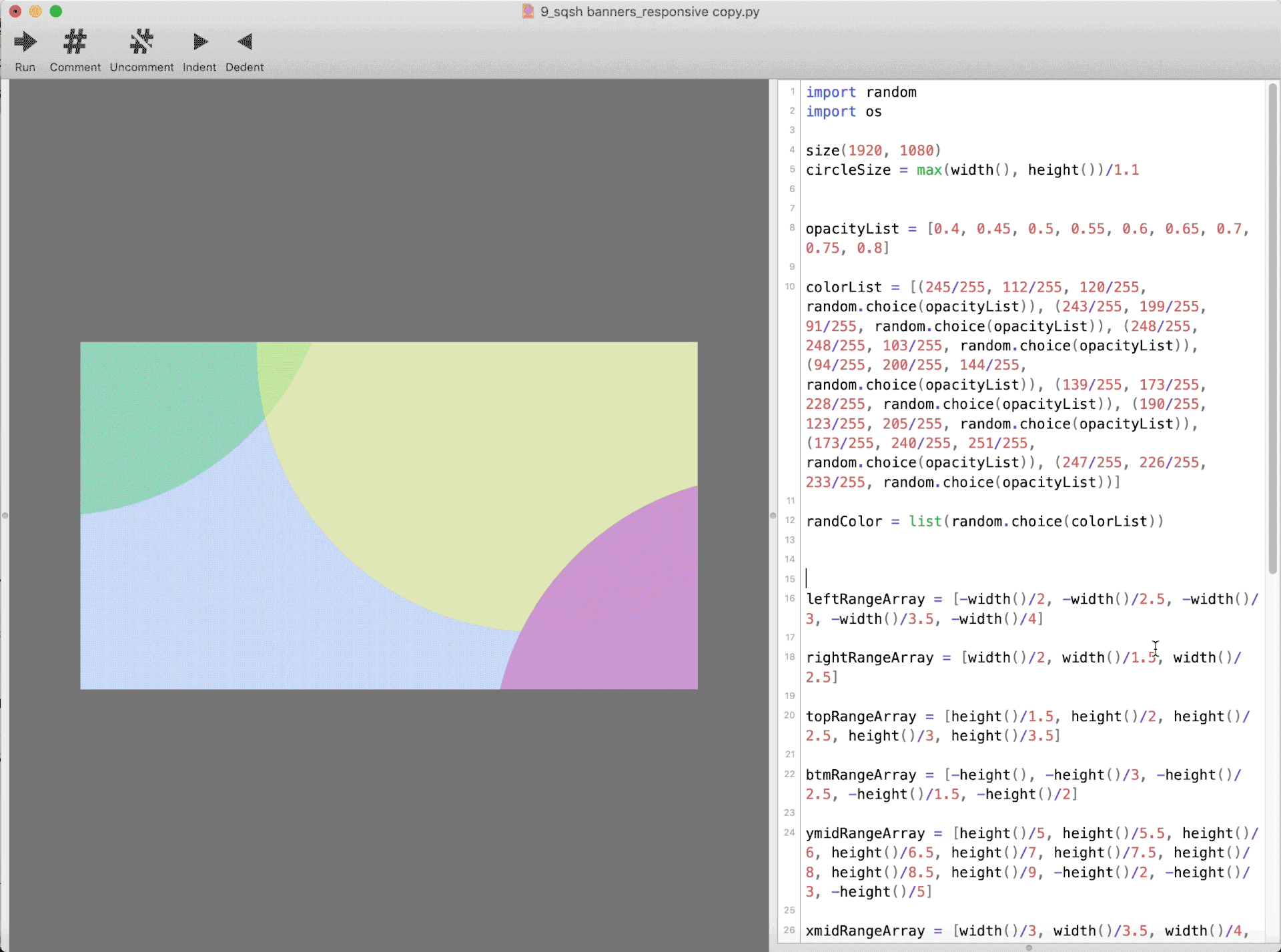
The SQSH, or St. Louis Queer+ Support Helpline, is a project started by Luka Cai, a friend and WashU alum, which aims to support the St. Louis LGBTQIA community by providing “free, confidential, and identity-affirming emotional support, resources, and referrals.” During the Summer of 2020, I worked with the SQSH web team to launch a revamp (made with Webflow) of the old site (made with Google Sites). You can read about the revamp process here!
When questioning how we could create visuals for the new website that would be exciting but easily made, I wondered if I could create code to generate simple yet widely varying backgrounds using Python in DrawBot. This would allow us to avoid using photos of people involved in SQSH, which can be time-consuming to photograph and maintain. But especially when shared publicly by an LGBTQ+ organization, photos of people can potentially endanger those people’s privacy and potentially out them to unwanted audiences.
Creating a background generator that anyone can use (for a variety of purposes such as for the website, social media posts, and merchandise) served as an interesting but daunting task, so I’m grateful to my mentor Ben Kiel for giving me tips along the way.
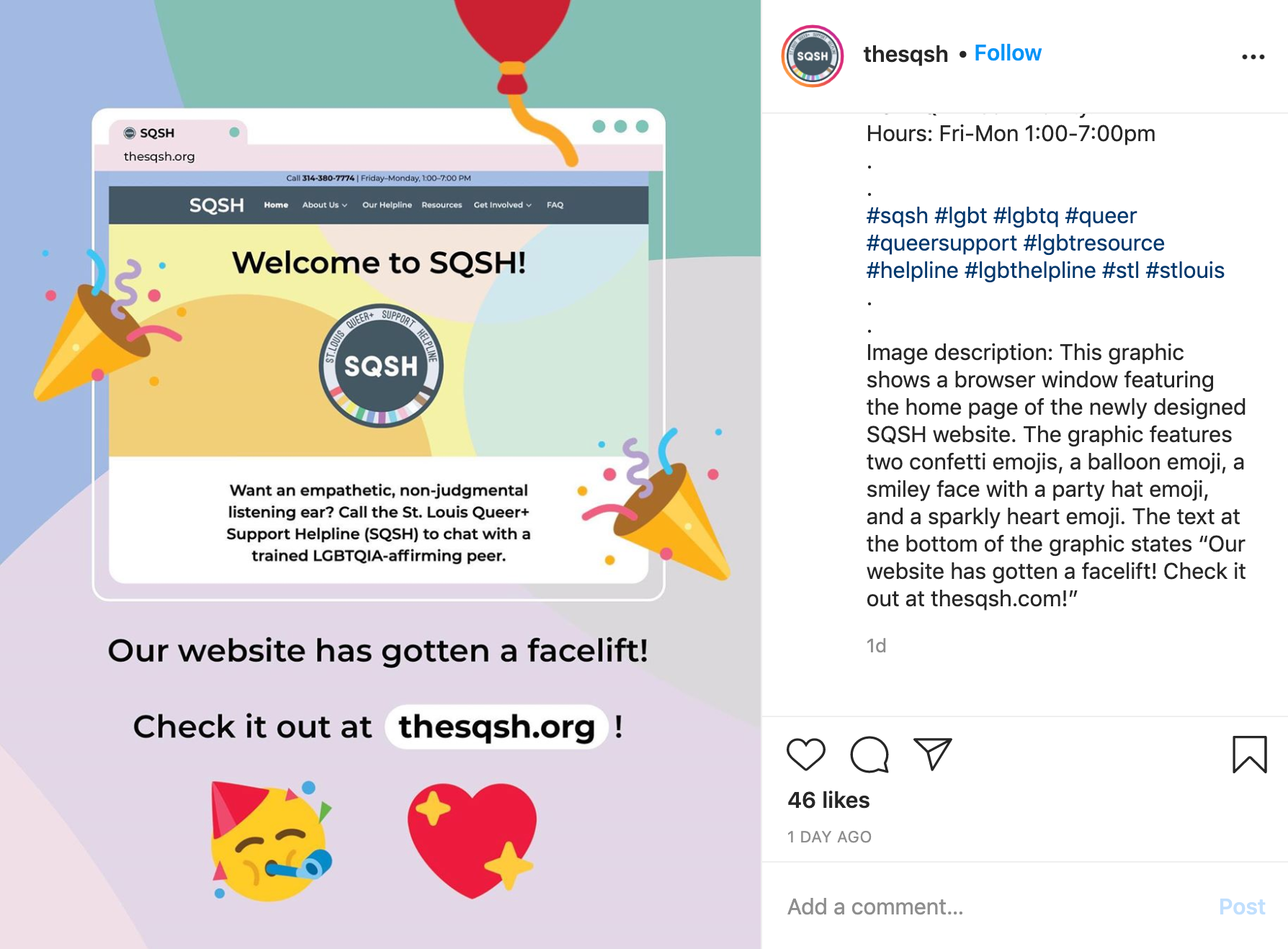
I was concurrently taking Ben Kiel's Programming Design course, in which we created visuals using Python in DrawBot. Inspired by designers like Andy Clymer and Agyei Archer, I wanted to see if I could utilize DrawBot to create an infinitely-varying yet similar set of graphics to use as backgrounds or images on the site.
Using DrawBot, I wrote code that generated 3 circles in random places on the canvas in 3 randomly-chosen colors (from the SQSH logo) in randomly-chosen opacities, with a background of 1 randomly-chosen color in a randomly-chosen opacity. I was attempting to evoke the round visuals of the SQSH business card.
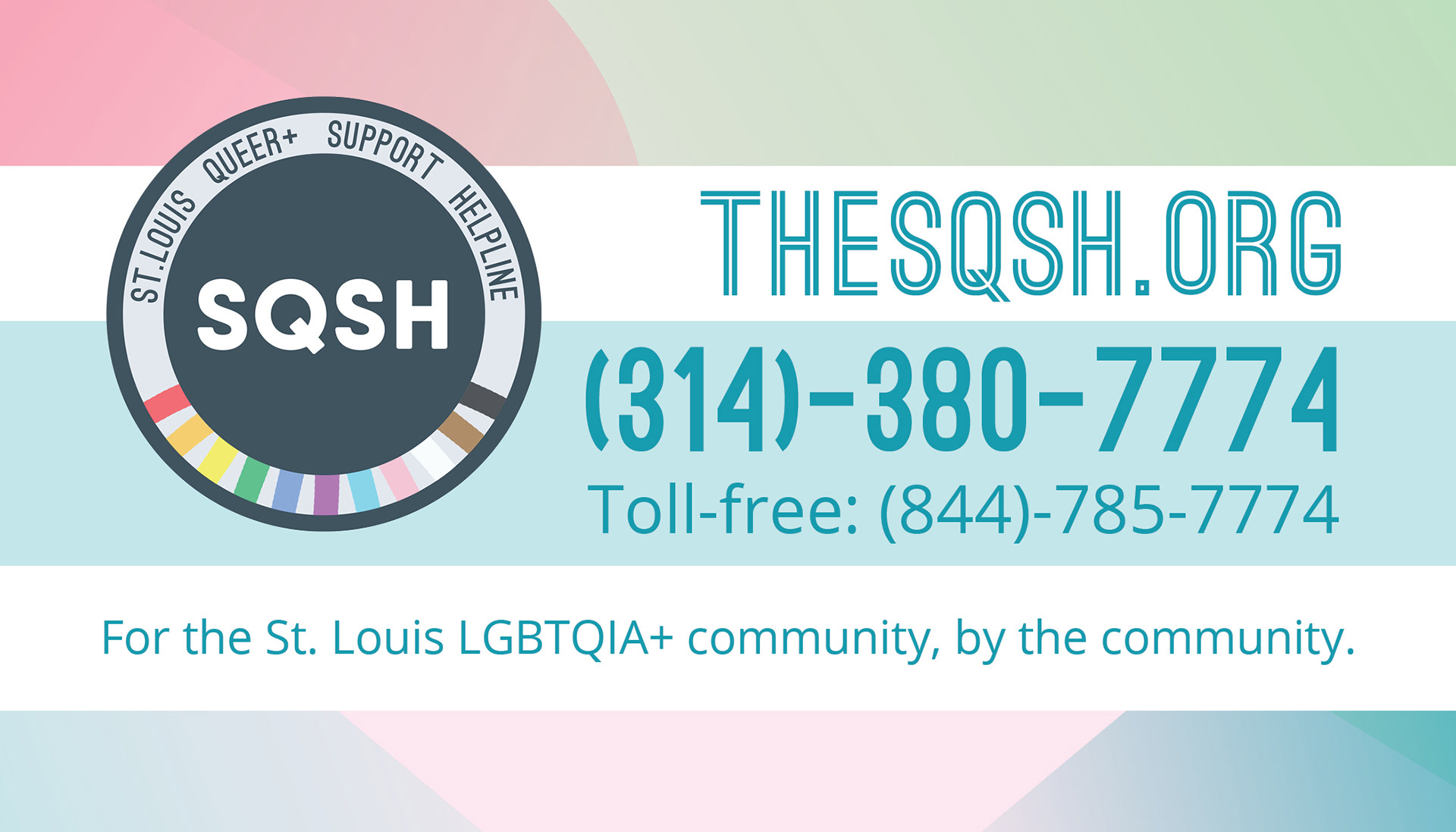
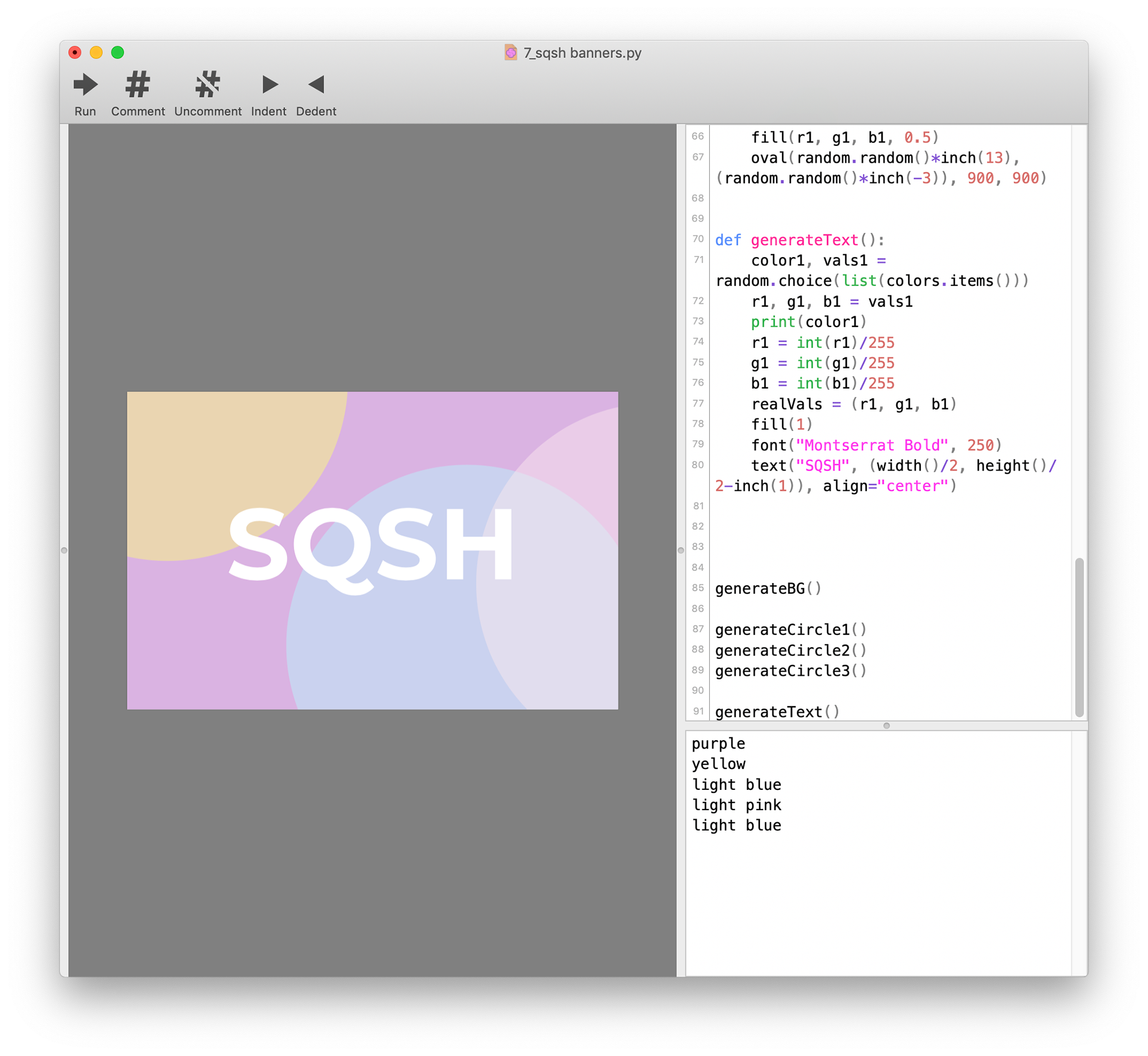
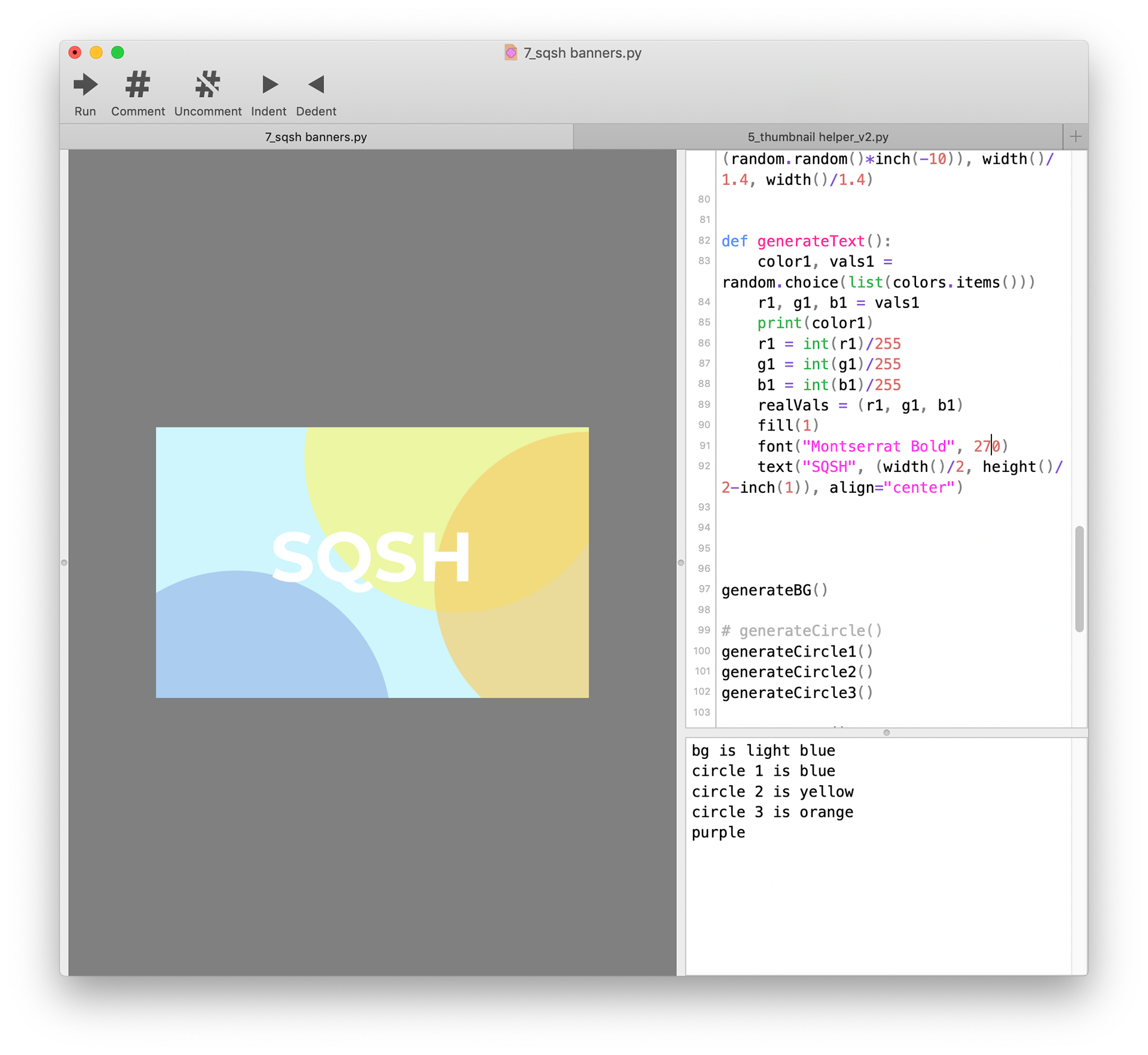
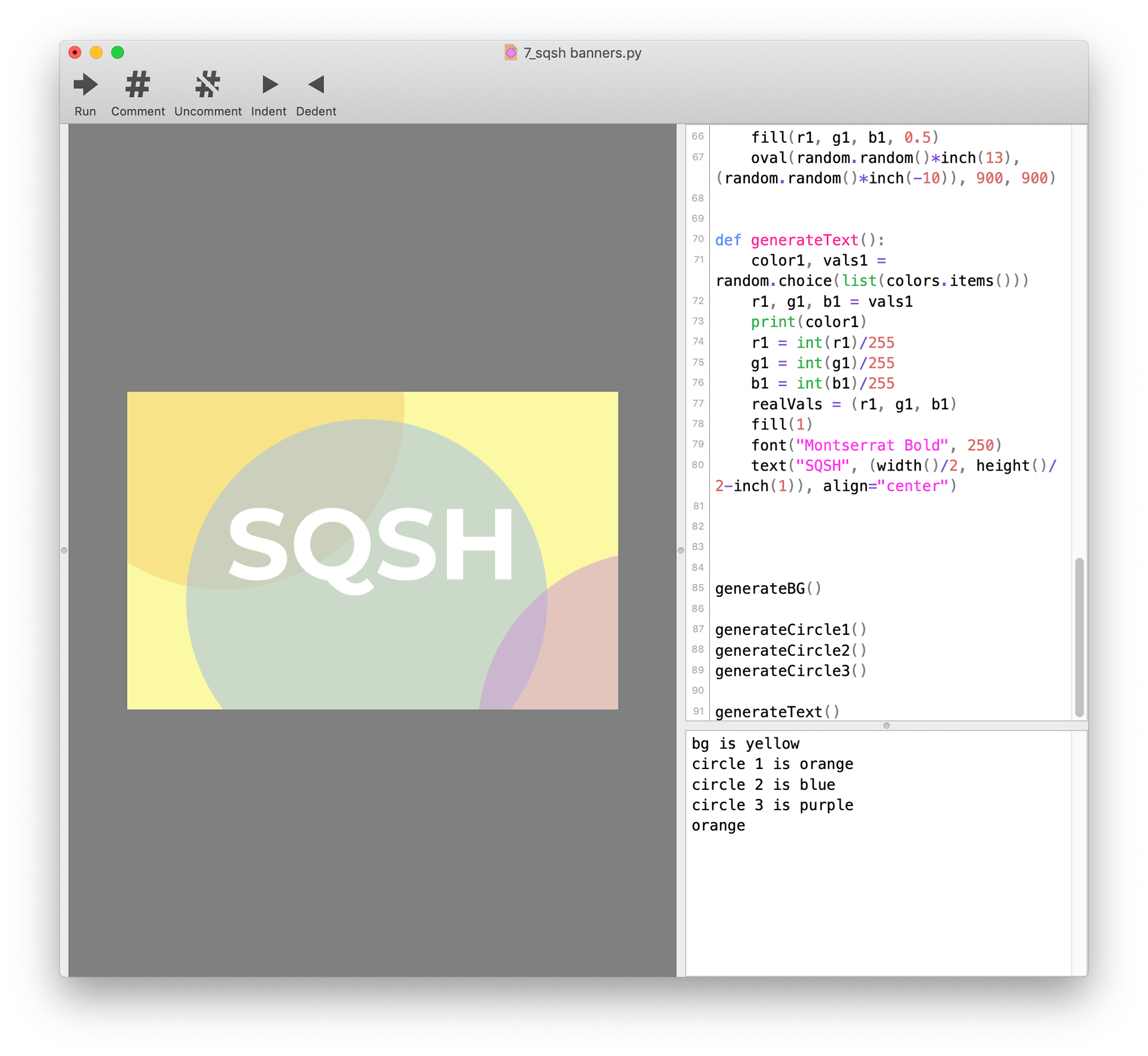
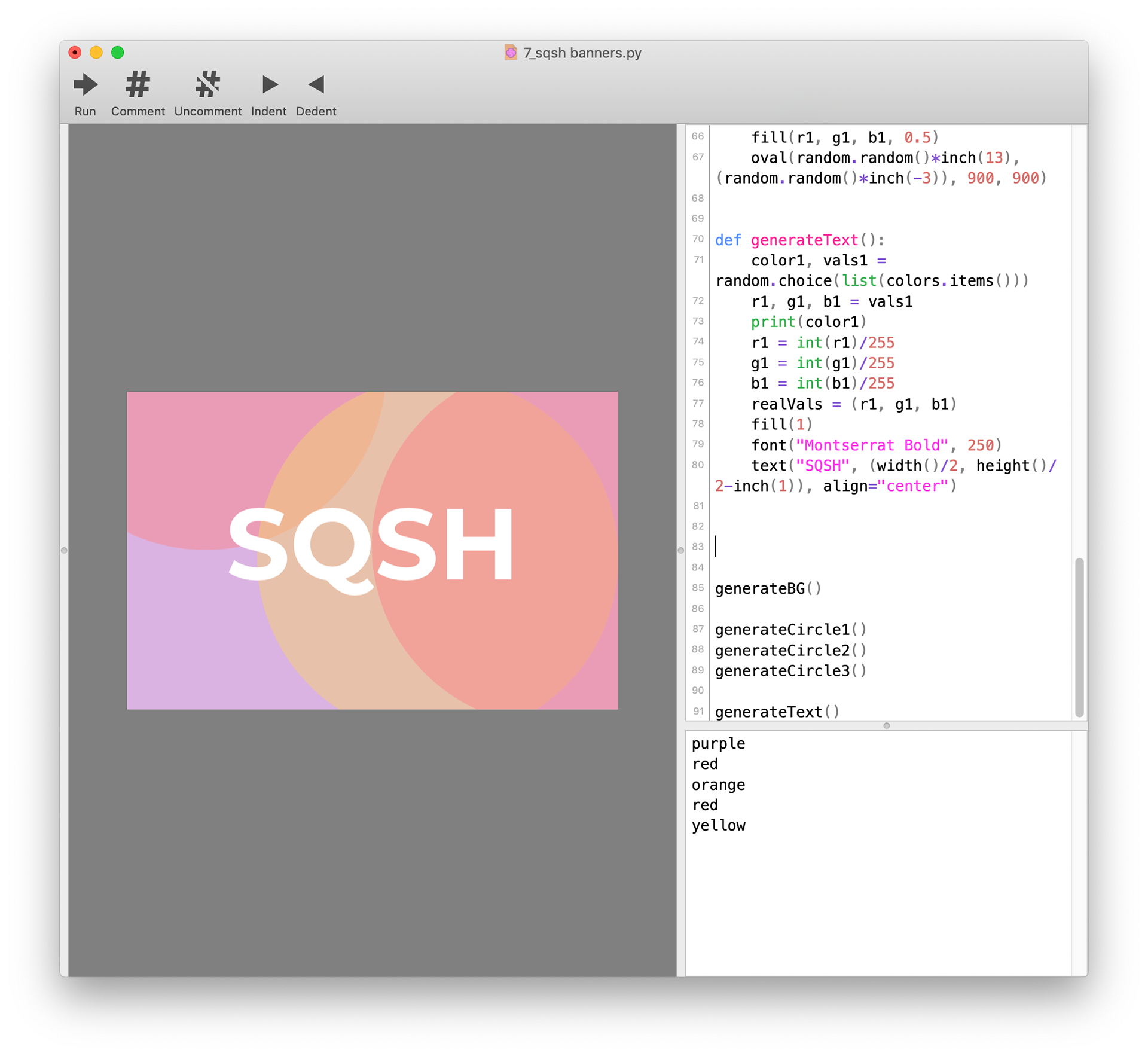
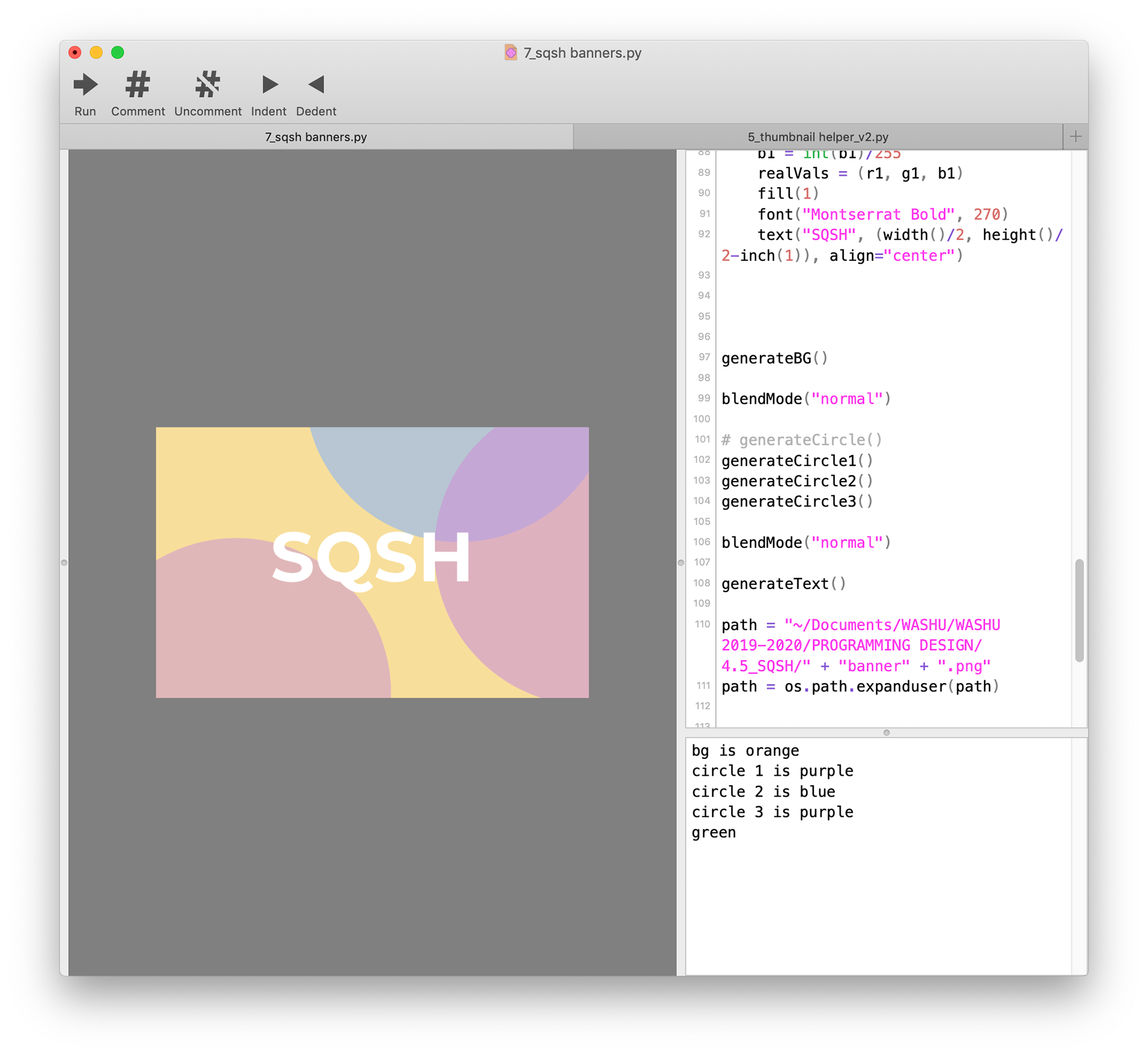
In later versions, I decided to get rid of the “SQSH” text because I wanted these to function purely as backgrounds.
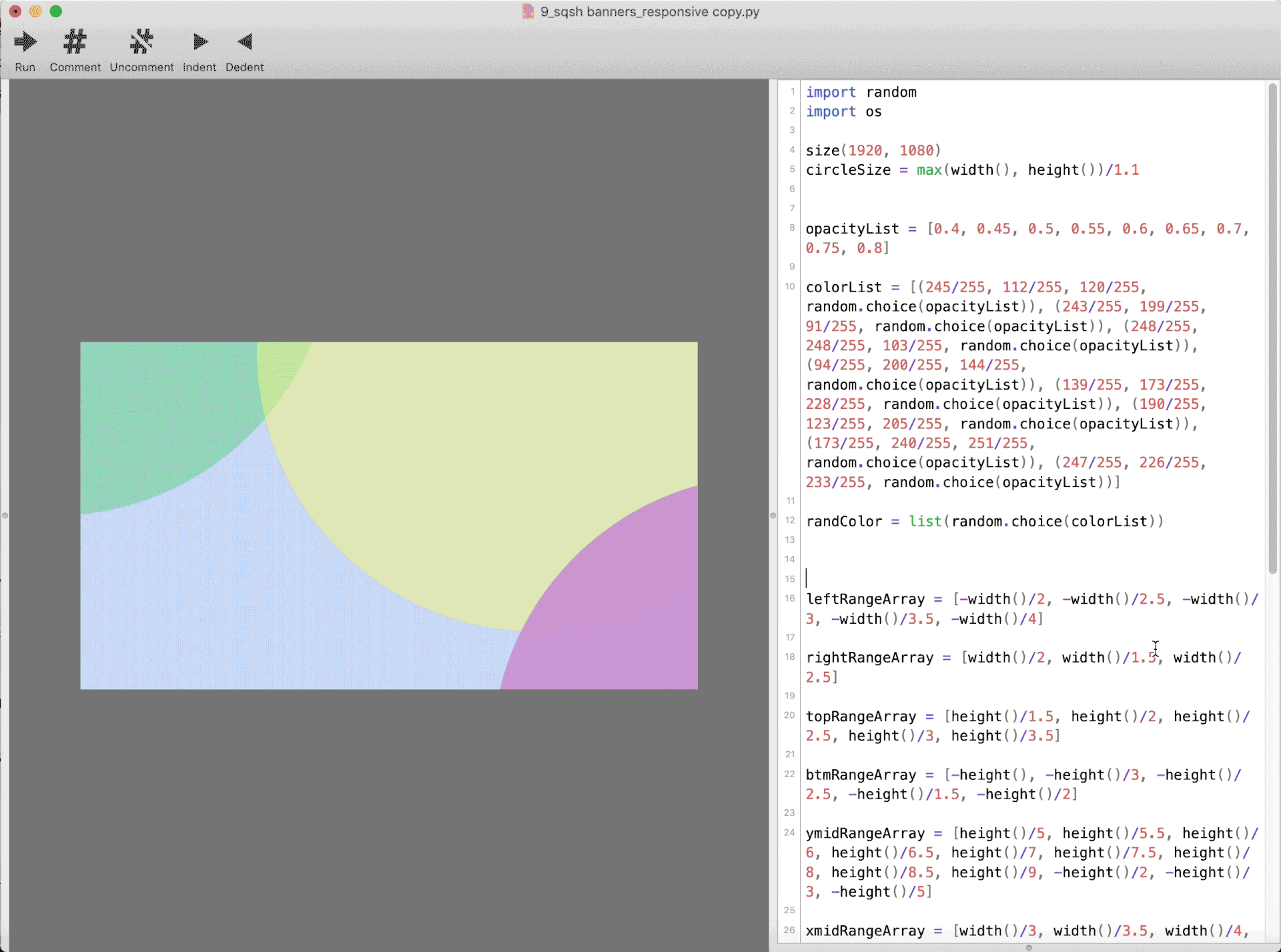
After generating many backgrounds, I put some on my website, one for each page’s header, one for a “get in touch” section at the bottom of each page, and others for any additional content that needed a background.
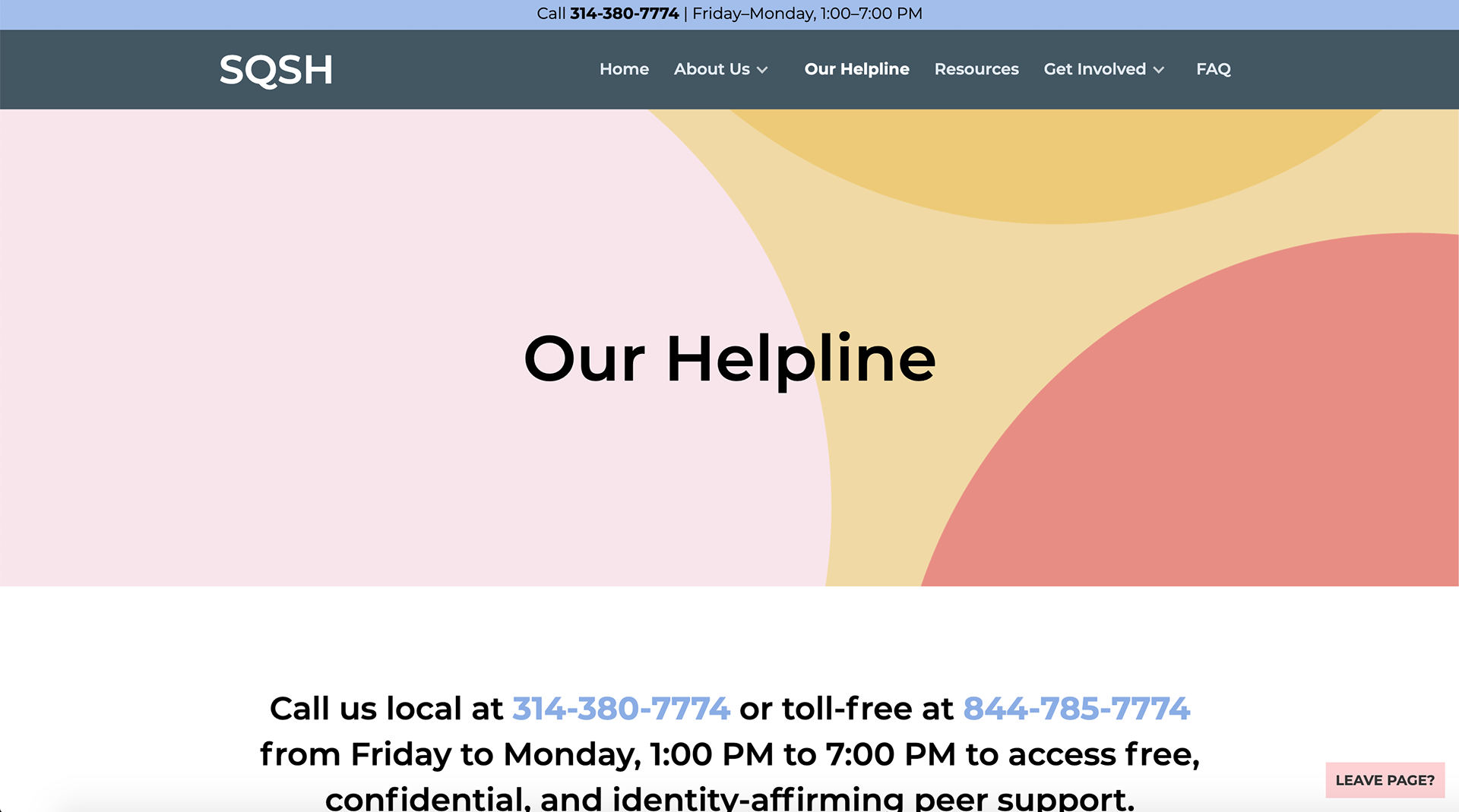
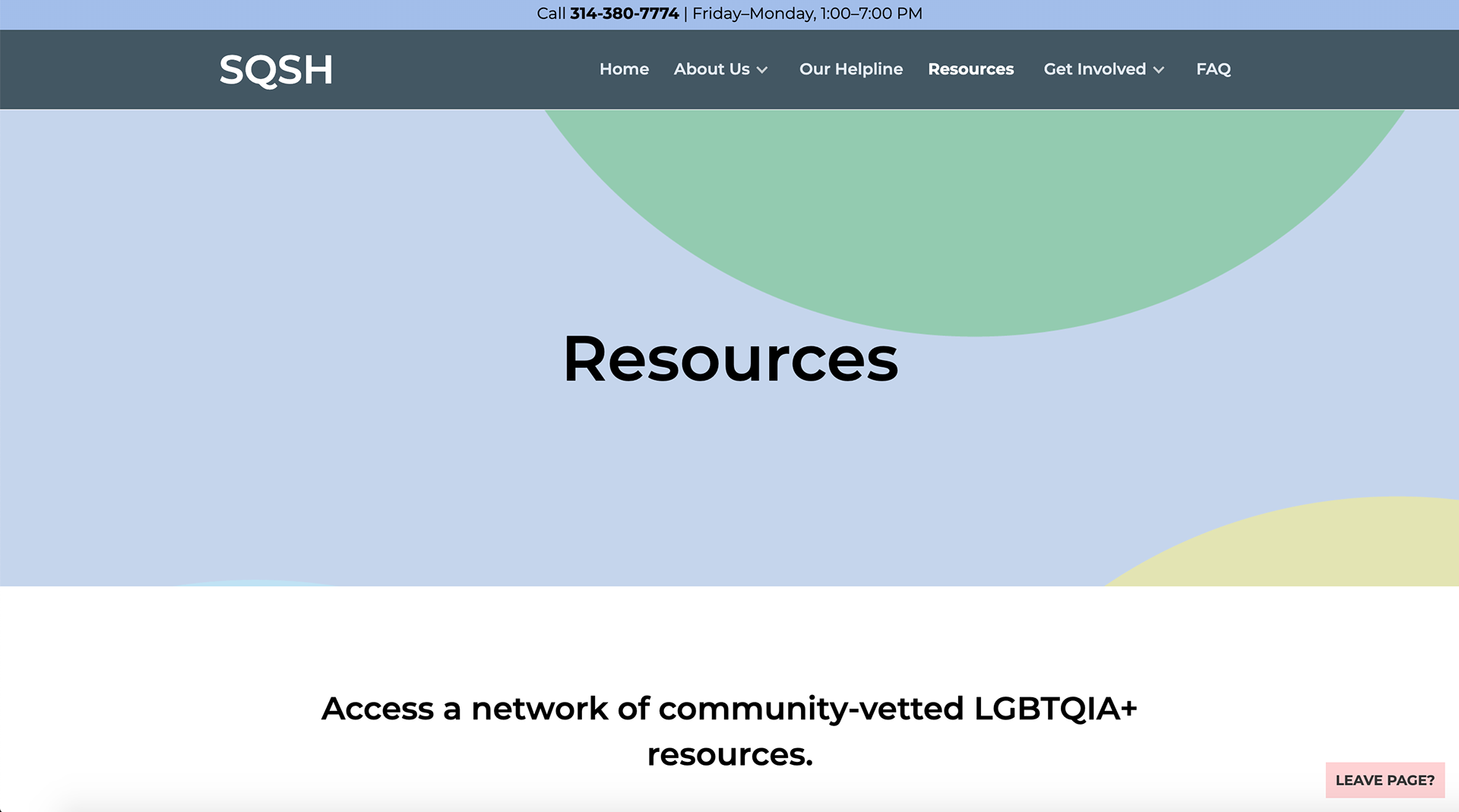
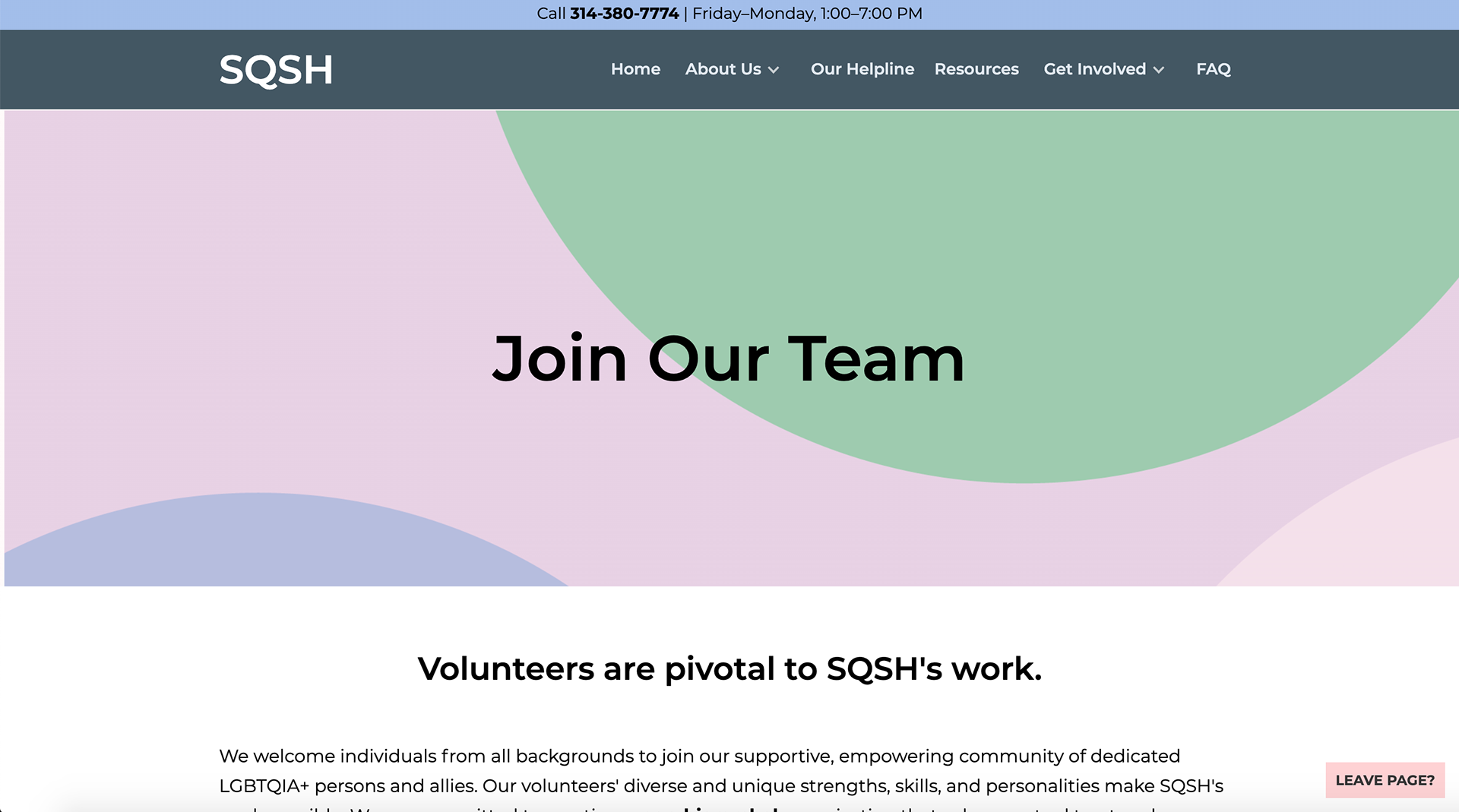
I began thinking I could expand these backgrounds to be used for social media as well, such as Facebook event headers and Instagram posts. I pitched this to the web team, who then asked me to pitch it to the social media team, who were fans of the idea. I set to work on creating an interface that would let others run my program without actually seeing any code.
I previously hard-coded numeric values to act as the widths and heights of the background and circles, but realized that if I wanted these to be used for multiple purposes, I’d have to make the circle sizes dependent on the background size.
The interface allows the user (likely someone on the SQSH social media team) to select different canvas sizes based on the background's use: Facebook header, Facebook event, Instagram square, Instagram story, and website banner. Once the user clicks "Generate," they can save the background as a PDF, PNG, or SVG to their computer.
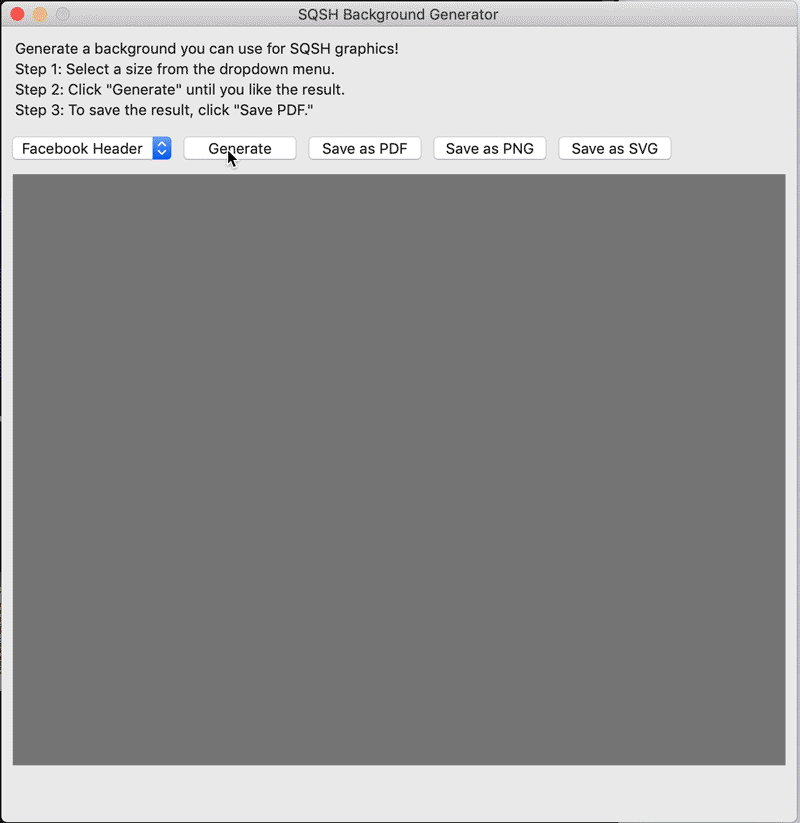
def generateBG():
fill(*randColor)
rect(0, 0, width(), height())
def generateCircle1():
color1, vals1 = random.choice(list(colors.items()))
r1, g1, b1 = vals1
print("circle 1 is " + color1)
r1 = int(r1)/255
g1 = int(g1)/255
b1 = int(b1)/255
realVals = (r1, g1, b1)
fill(r1, g1, b1, random.choice(opacityList))
oval(random.choice(leftRangeArray), random.choice(topRangeArray), circleSize, circleSize)
def generateCircle2():
color1, vals1 = random.choice(list(colors.items()))
r1, g1, b1 = vals1
print("circle 2 is " + color1)
r1 = int(r1)/255
g1 = int(g1)/255
b1 = int(b1)/255
fill(r1, g1, b1, random.choice(opacityList))
oval(random.choice(rightRangeArray), random.choice(btmRangeArray), circleSize, circleSize)
def generateCircle3():
color1, vals1 = random.choice(list(colors.items()))
r1, g1, b1 = vals1
print("circle 3 is " + color1)
r1 = int(r1)/255
g1 = int(g1)/255
b1 = int(b1)/255
realVals = (r1, g1, b1)
fill(r1, g1, b1, random.choice(opacityList))
oval(random.choice(xmidRangeArray), random.choice(ymidRangeArray), circleSize, circleSize)
generateBG()
generateCircle1()
generateCircle2()
generateCircle3()
fill(*randColor)
rect(0, 0, width(), height())
def generateCircle1():
color1, vals1 = random.choice(list(colors.items()))
r1, g1, b1 = vals1
print("circle 1 is " + color1)
r1 = int(r1)/255
g1 = int(g1)/255
b1 = int(b1)/255
realVals = (r1, g1, b1)
fill(r1, g1, b1, random.choice(opacityList))
oval(random.choice(leftRangeArray), random.choice(topRangeArray), circleSize, circleSize)
def generateCircle2():
color1, vals1 = random.choice(list(colors.items()))
r1, g1, b1 = vals1
print("circle 2 is " + color1)
r1 = int(r1)/255
g1 = int(g1)/255
b1 = int(b1)/255
fill(r1, g1, b1, random.choice(opacityList))
oval(random.choice(rightRangeArray), random.choice(btmRangeArray), circleSize, circleSize)
def generateCircle3():
color1, vals1 = random.choice(list(colors.items()))
r1, g1, b1 = vals1
print("circle 3 is " + color1)
r1 = int(r1)/255
g1 = int(g1)/255
b1 = int(b1)/255
realVals = (r1, g1, b1)
fill(r1, g1, b1, random.choice(opacityList))
oval(random.choice(xmidRangeArray), random.choice(ymidRangeArray), circleSize, circleSize)
generateBG()
generateCircle1()
generateCircle2()
generateCircle3()
In the near future, I'll work to get my background generator as a standalone Mac app, as well as do extensive user testing with other SQSH teams to make sure my generator is understandable and easily usable by others.
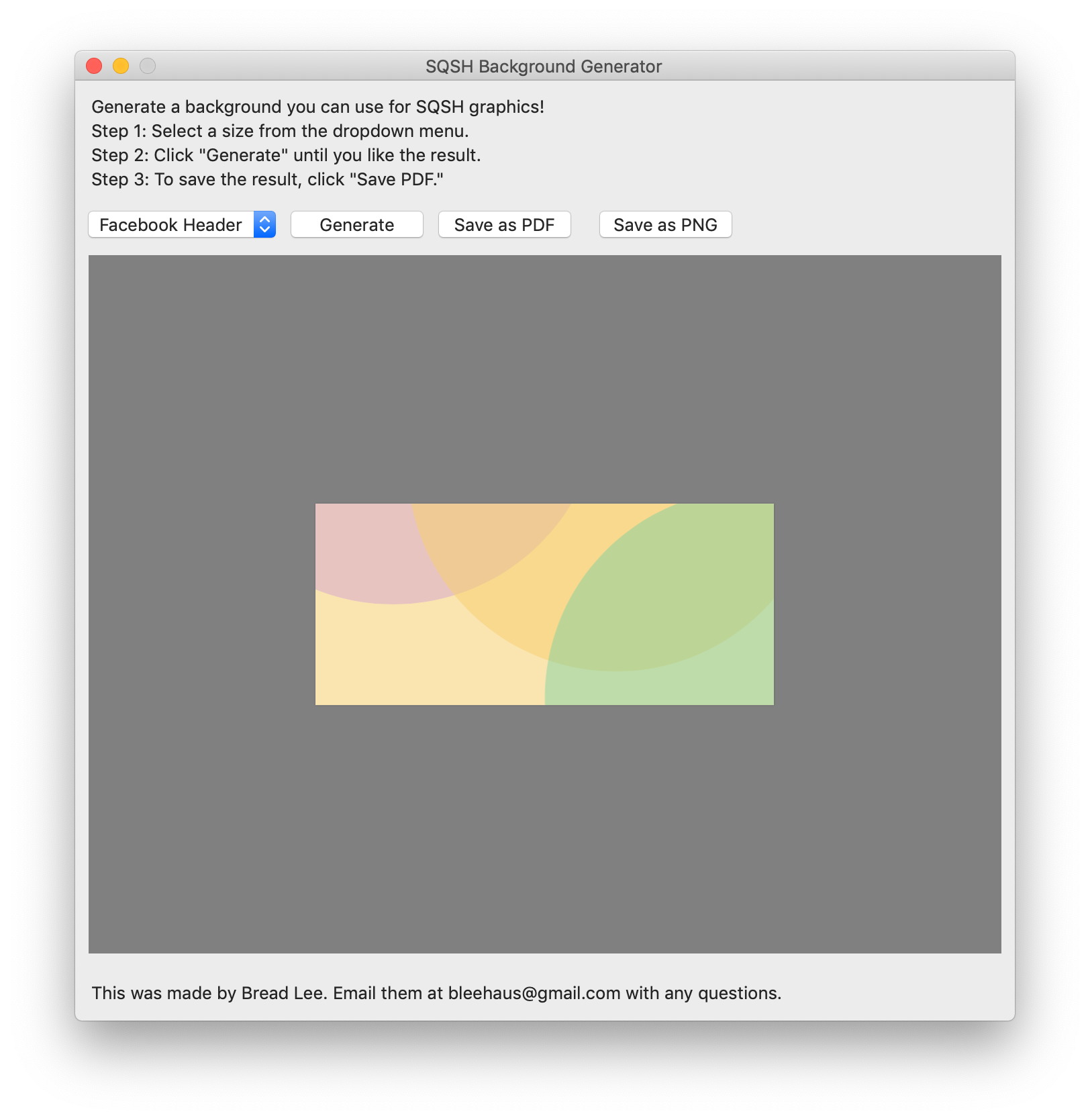
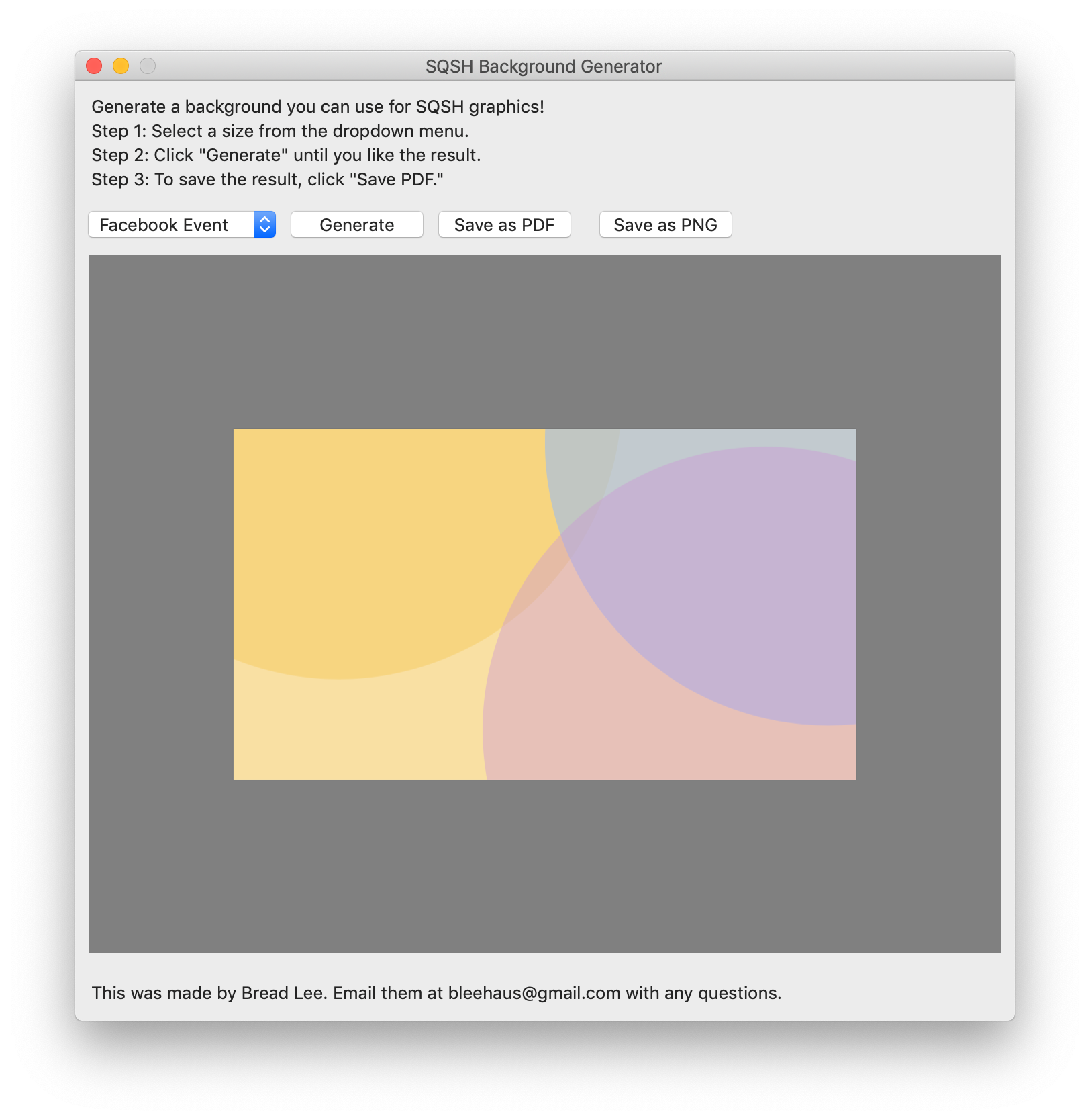
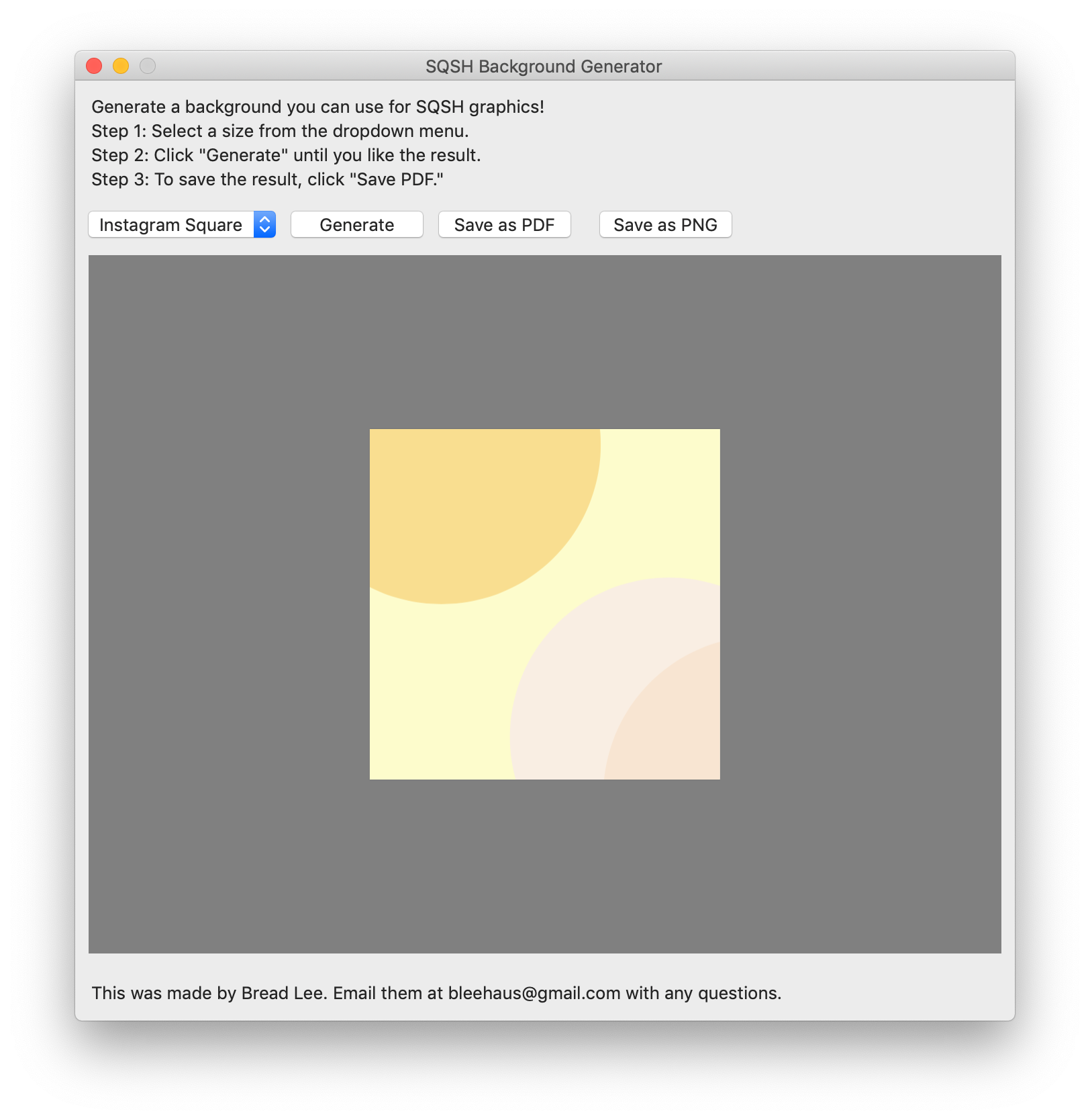
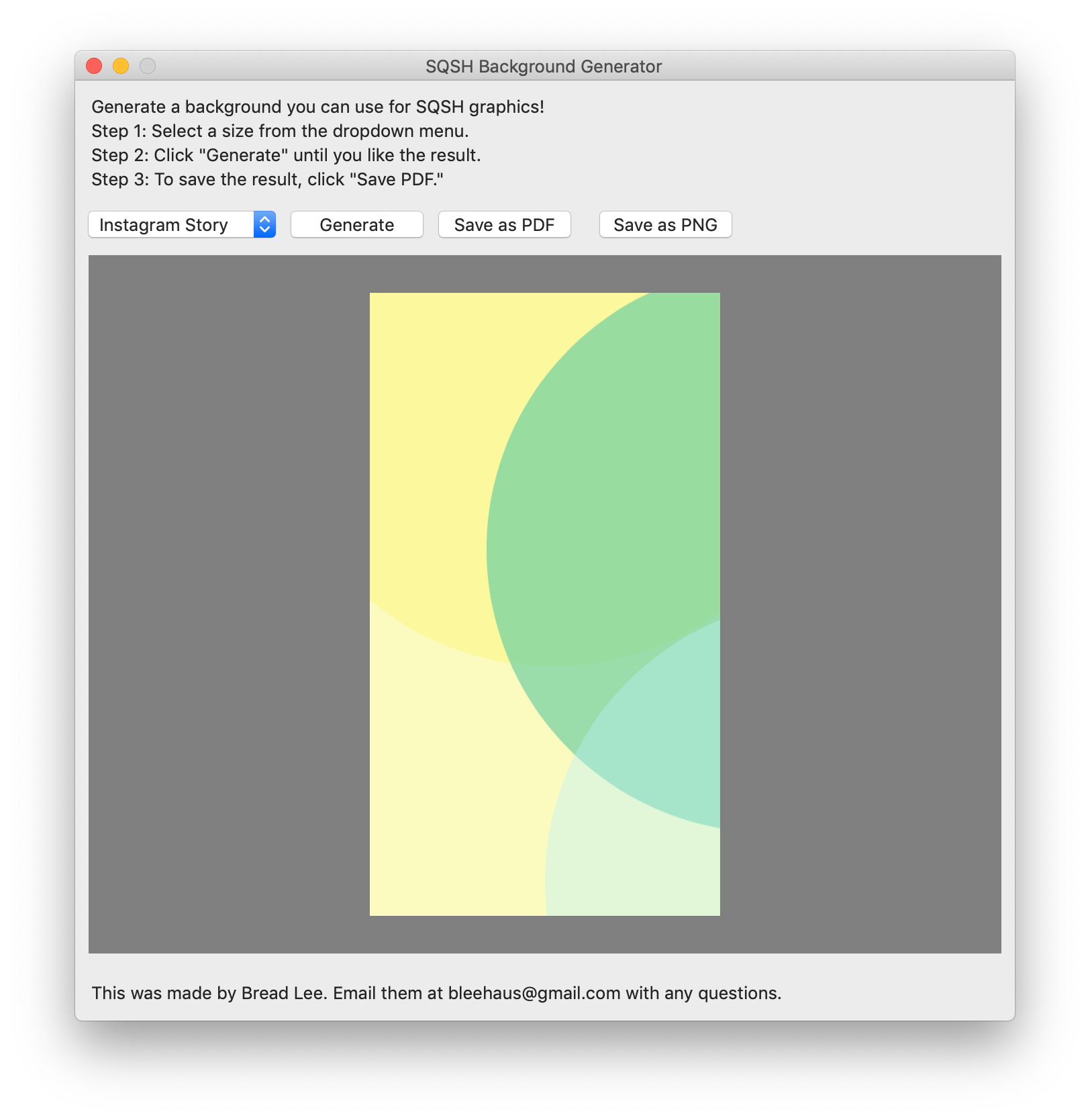
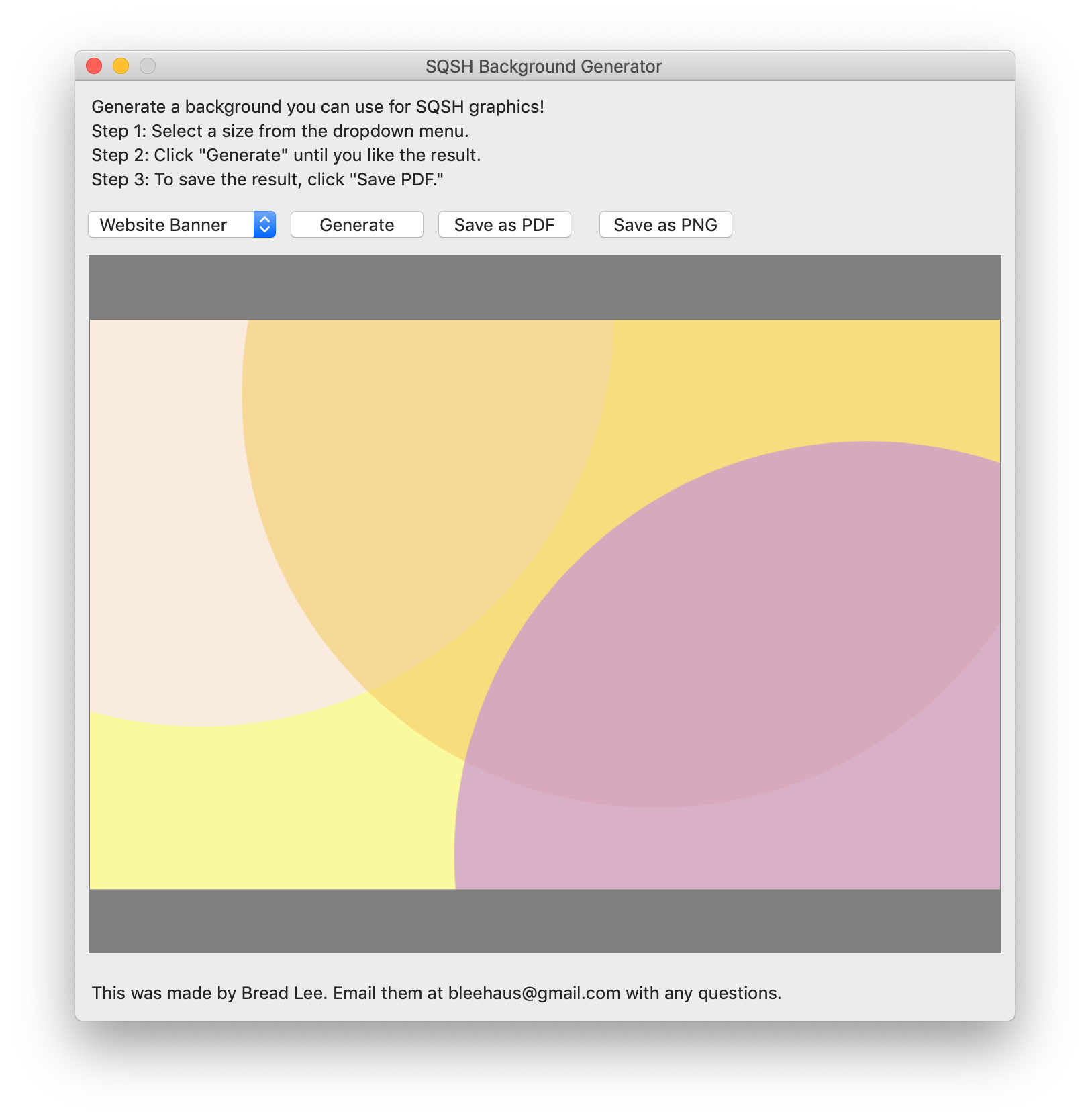
I’m excited to see how this generator can help the SQSH Social Media team!
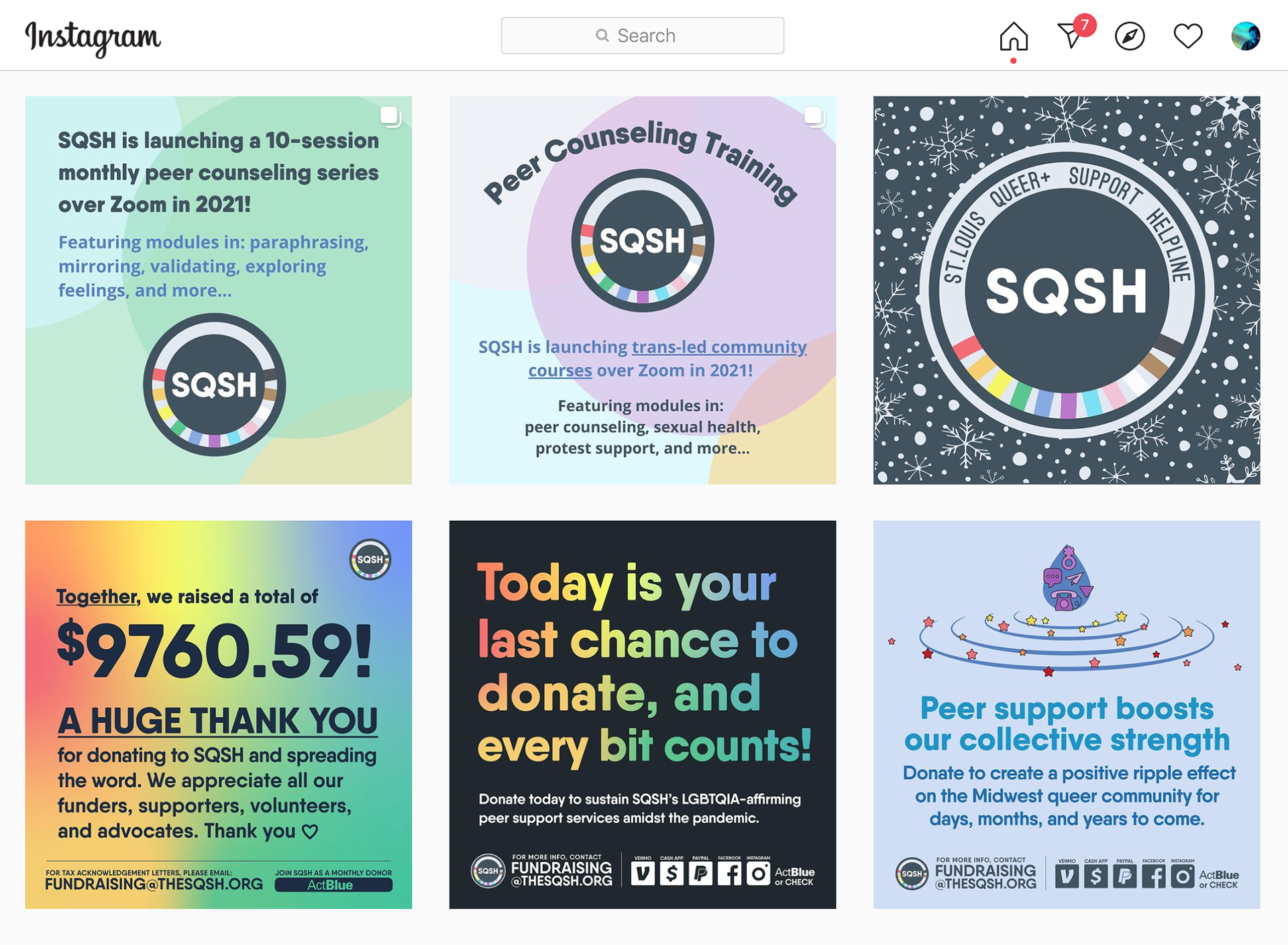
Update: As of January 2021, I’ve done a lot of reflecting and have noticed that when it comes to social media posts, the hard edges of the circles can distract from the content and overall make these harder to use as backgrounds. I realize my original intent for how these would be used probably felt restrictive to the SQSH Social Media Coordinator and Graphic Design Team. I took the Graphic Design Team’s use of gradients for social media posts in stride (and I’d already made some SQSH color gradients for the website’s dropdowns!). For the next batch of generated backgrounds, I made multiple version of each background: unedited, unedited gradient, blob, and blob gradient. My intention with the “blob” and both gradient versions is to clear out space in the middle so as to not distract from the content. I also handed over my generator (with instructions) to the Social Media Coordinator who, to my relief, was able to use it!
This experience has taught me that brand identities need to be viewed as living documents (to an extent, of course) and should allow for flexibility. As SQSH’s brand identity adapts, I hope to adapt along with it and foster its growth.
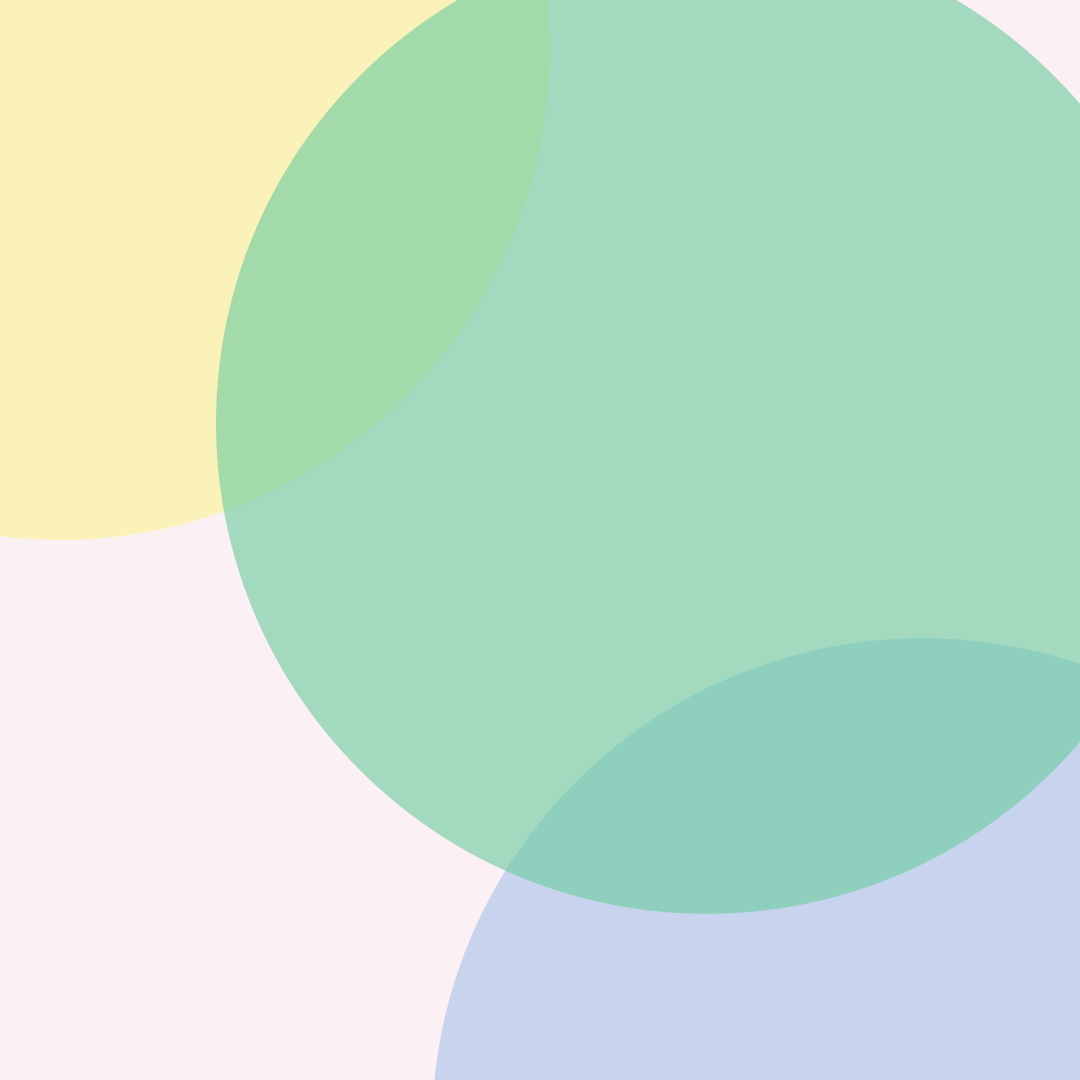
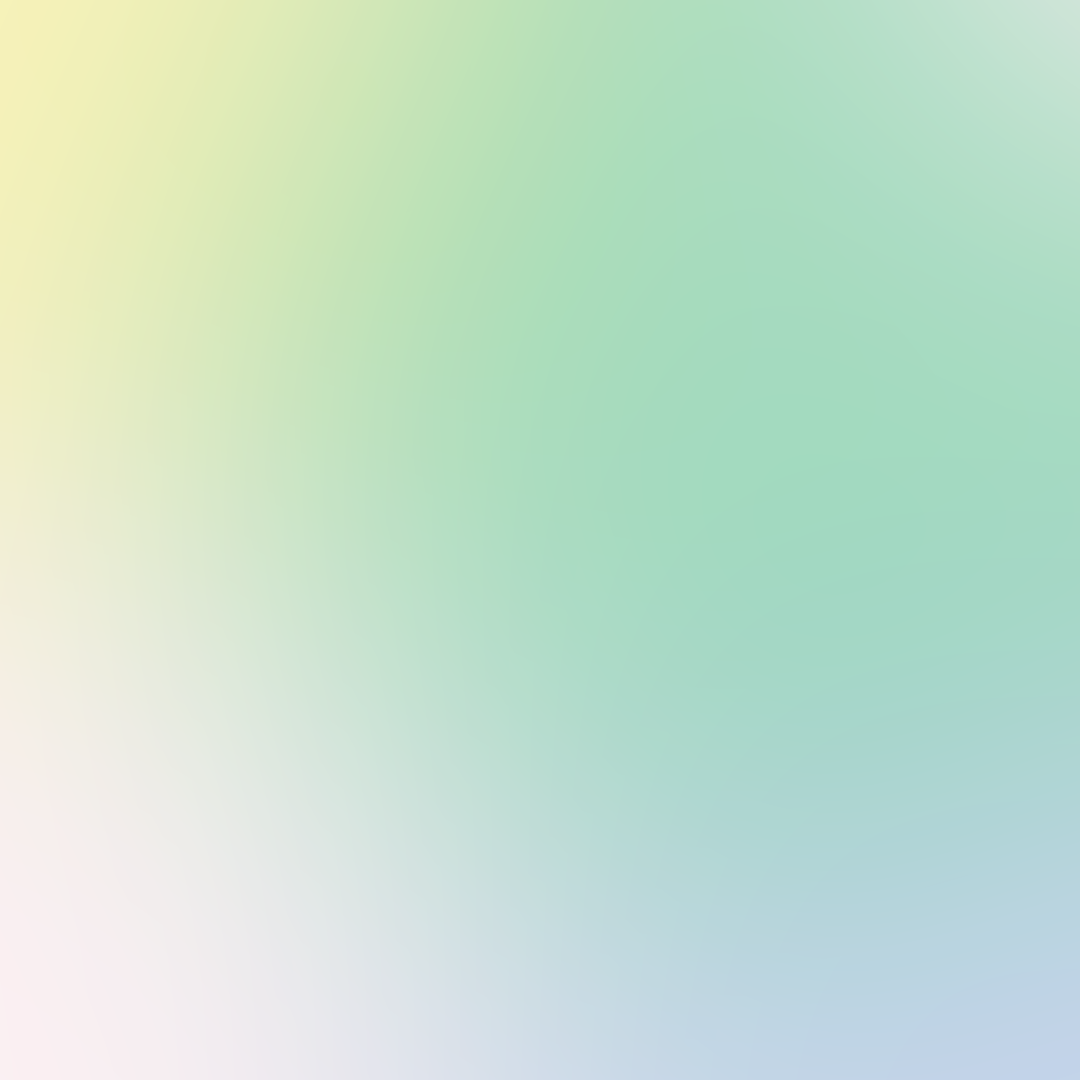
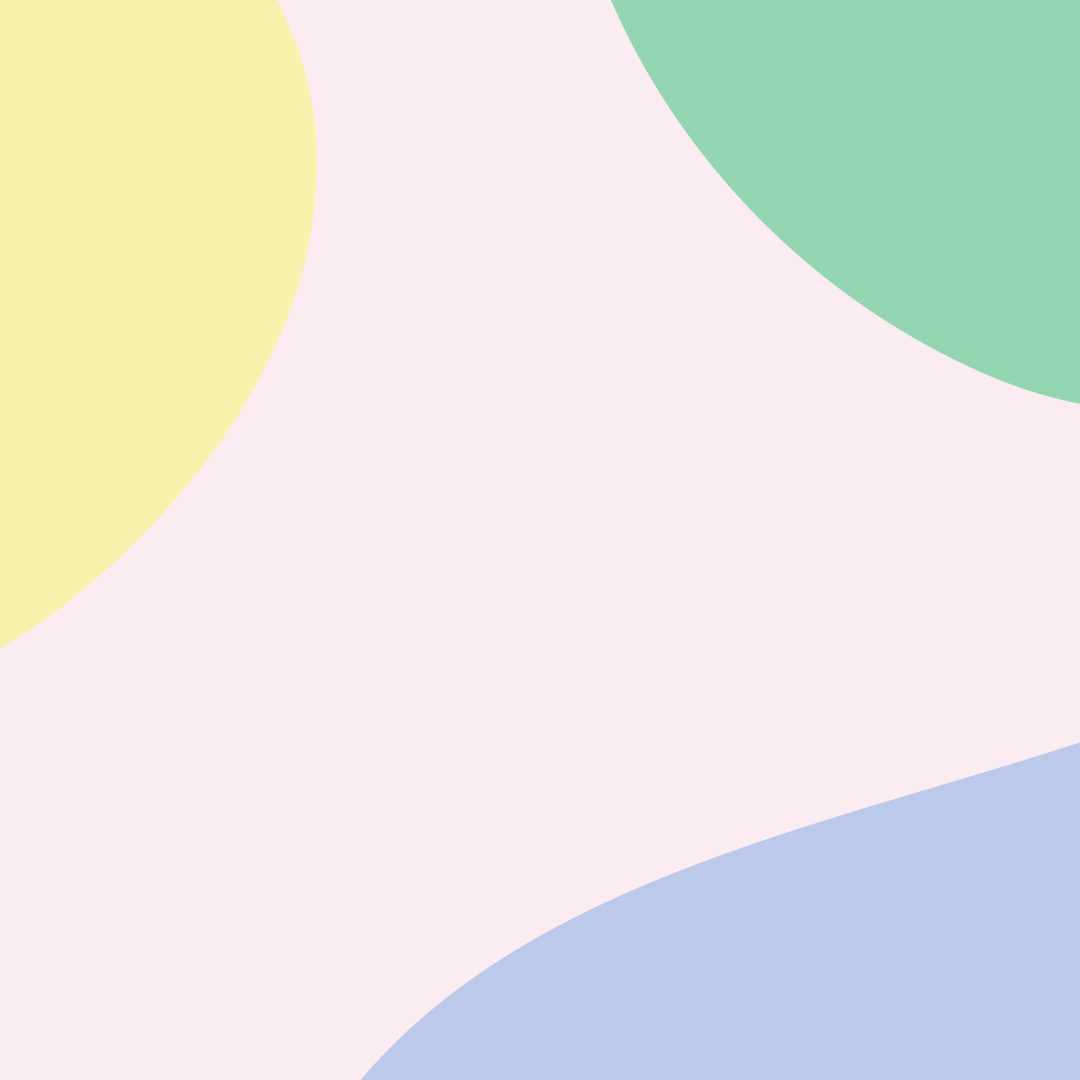
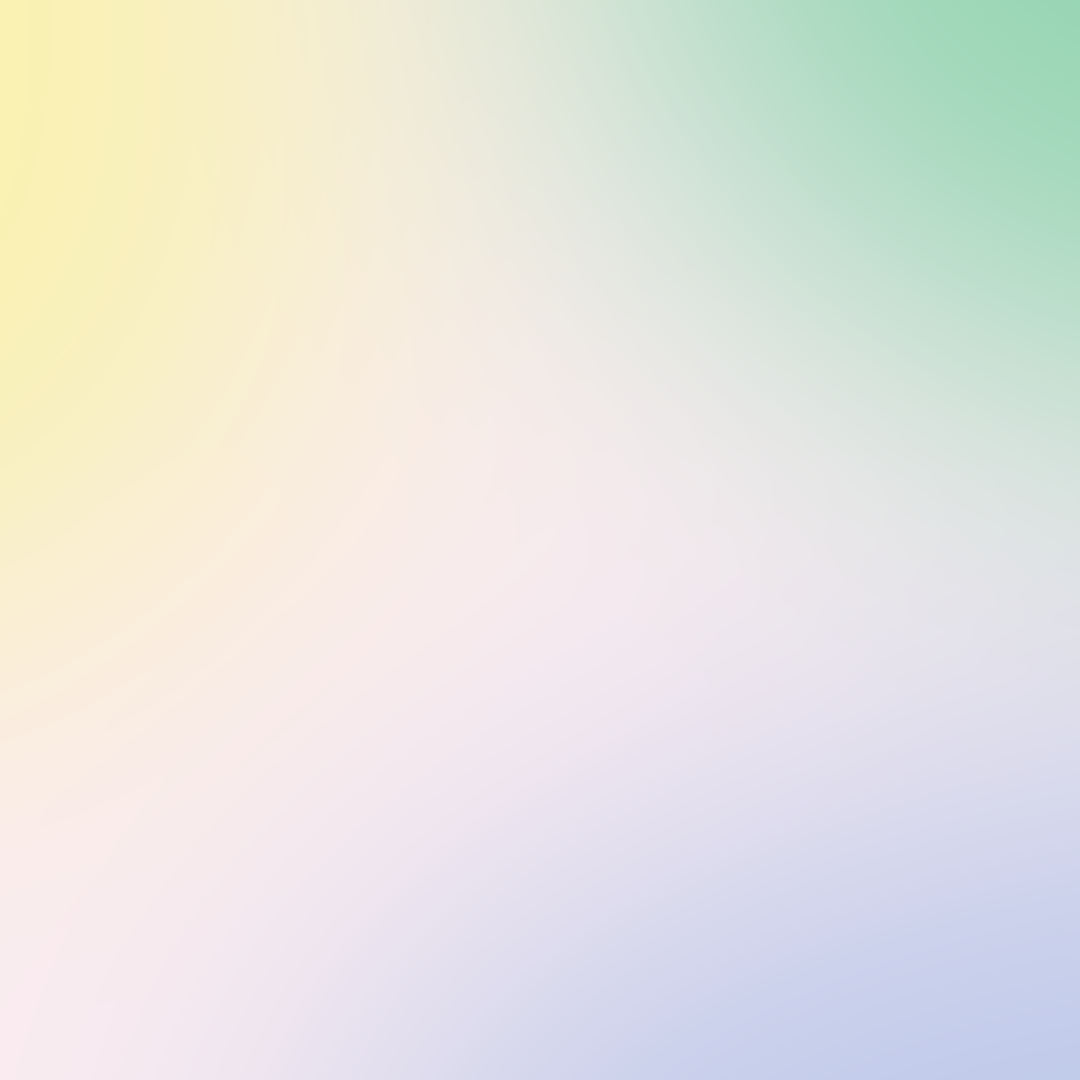
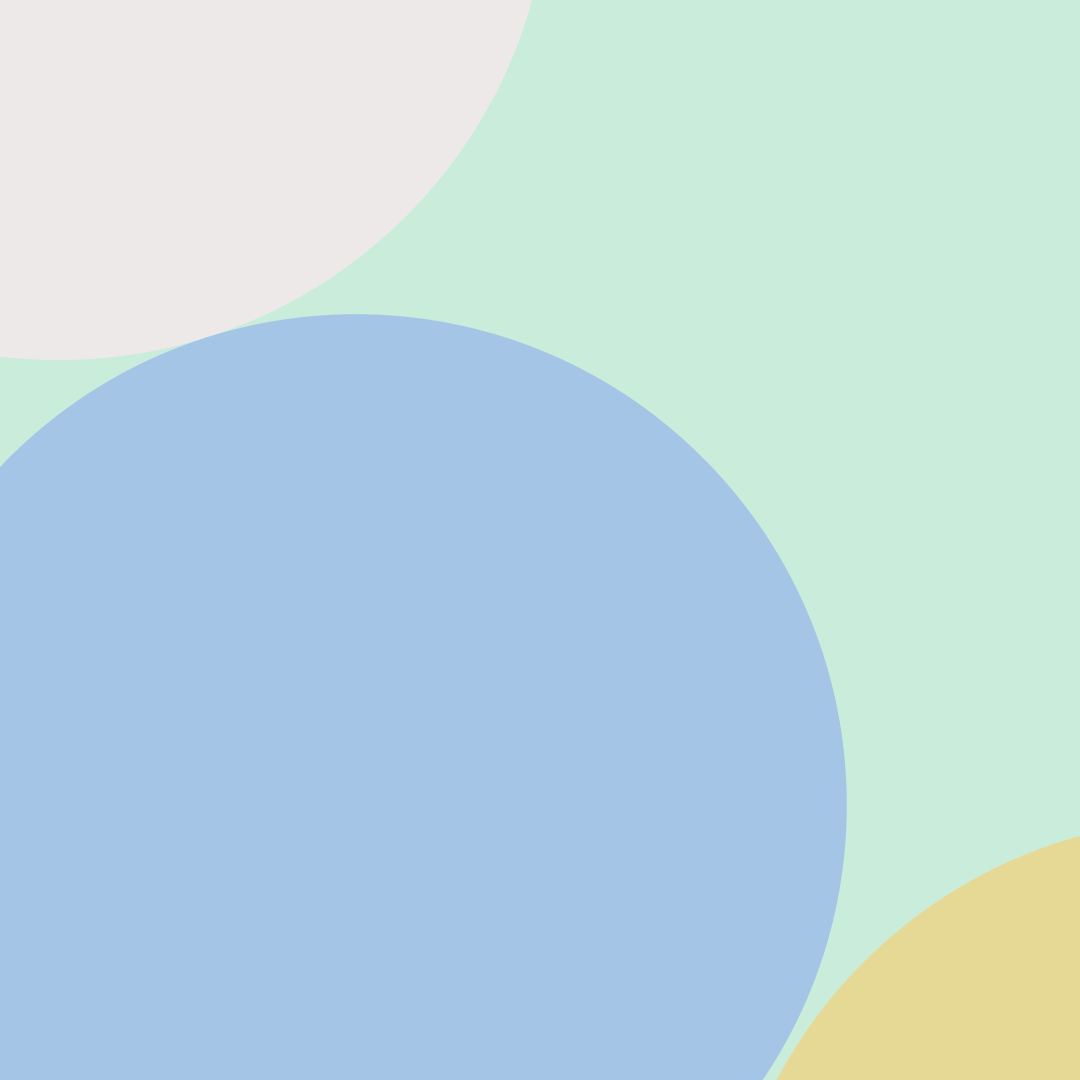
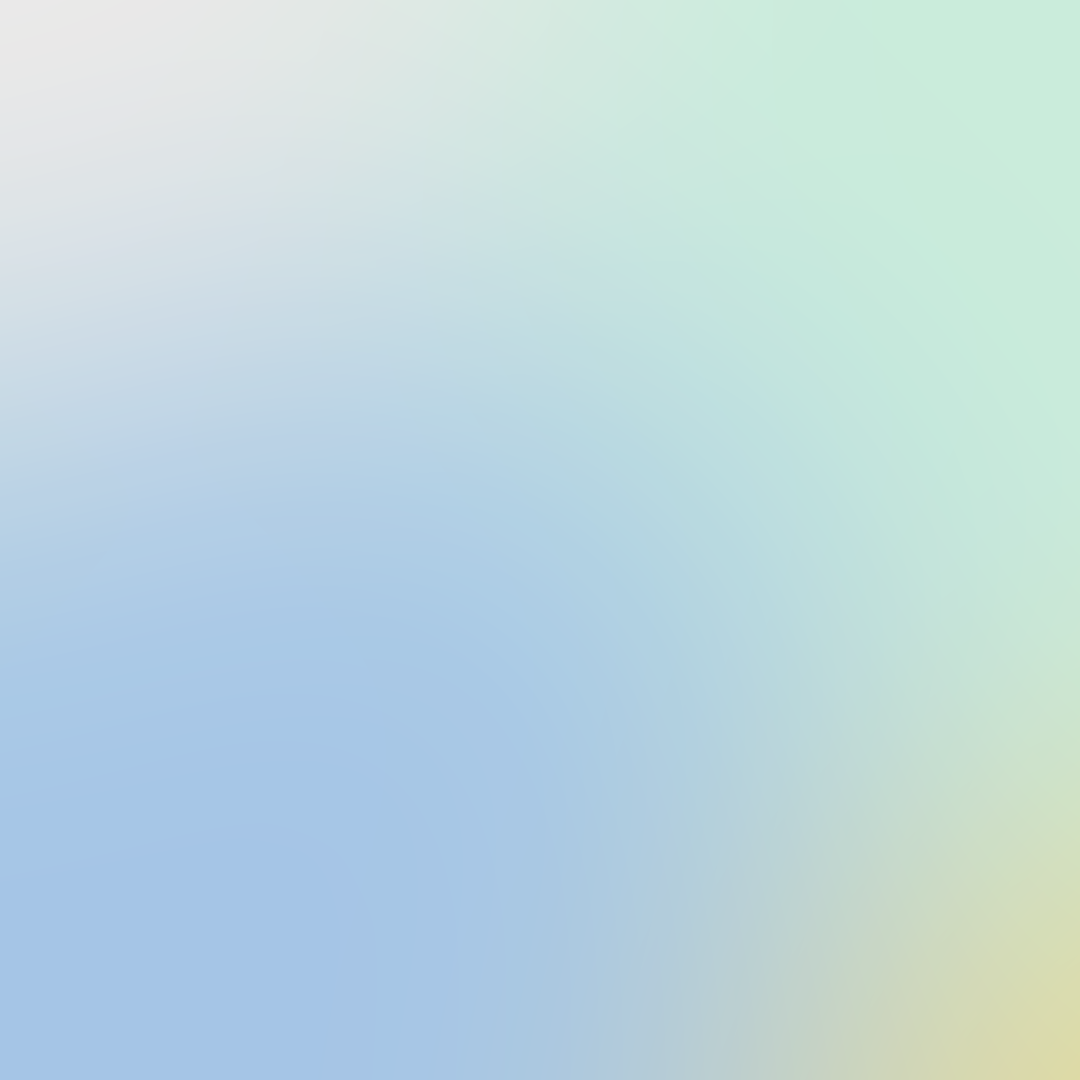
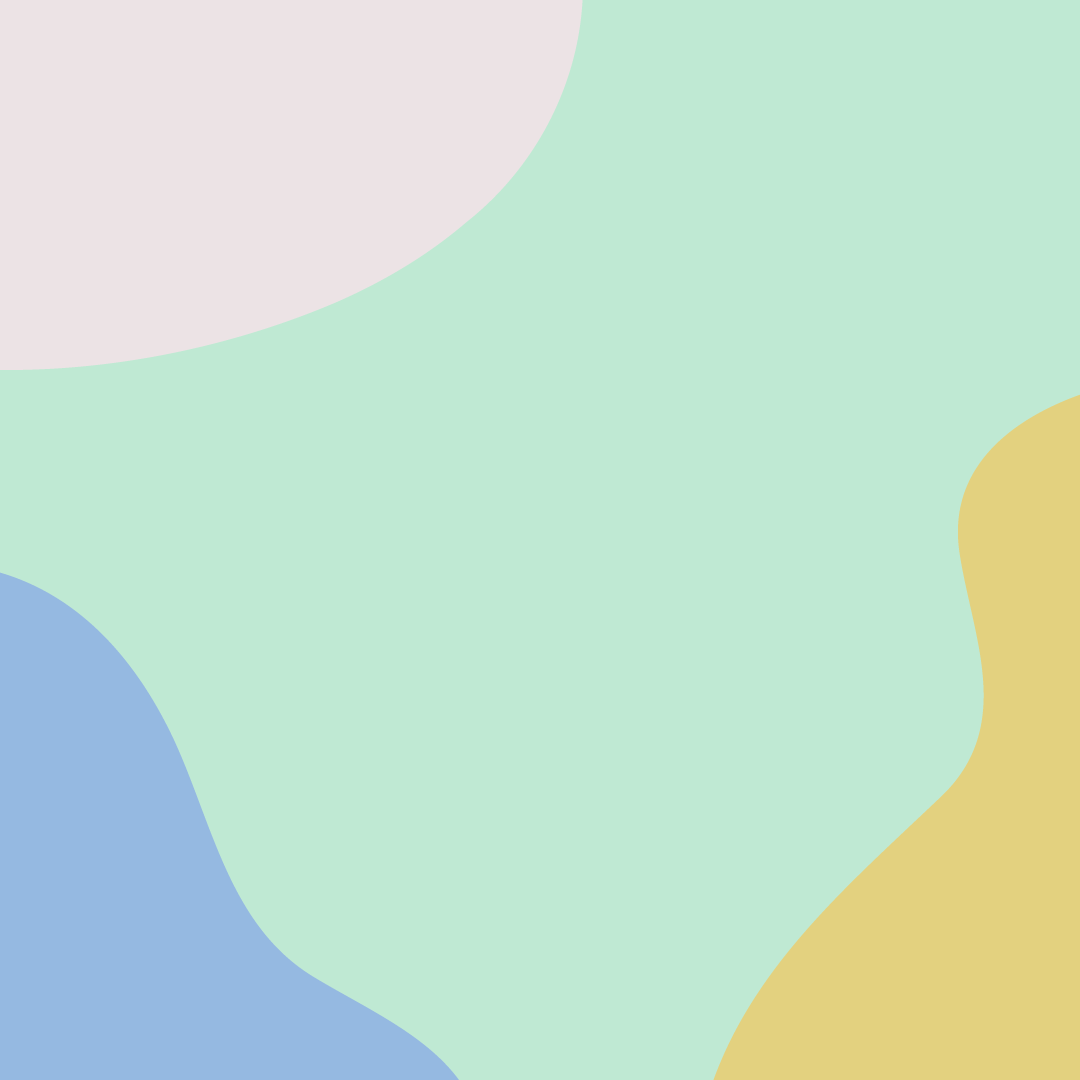
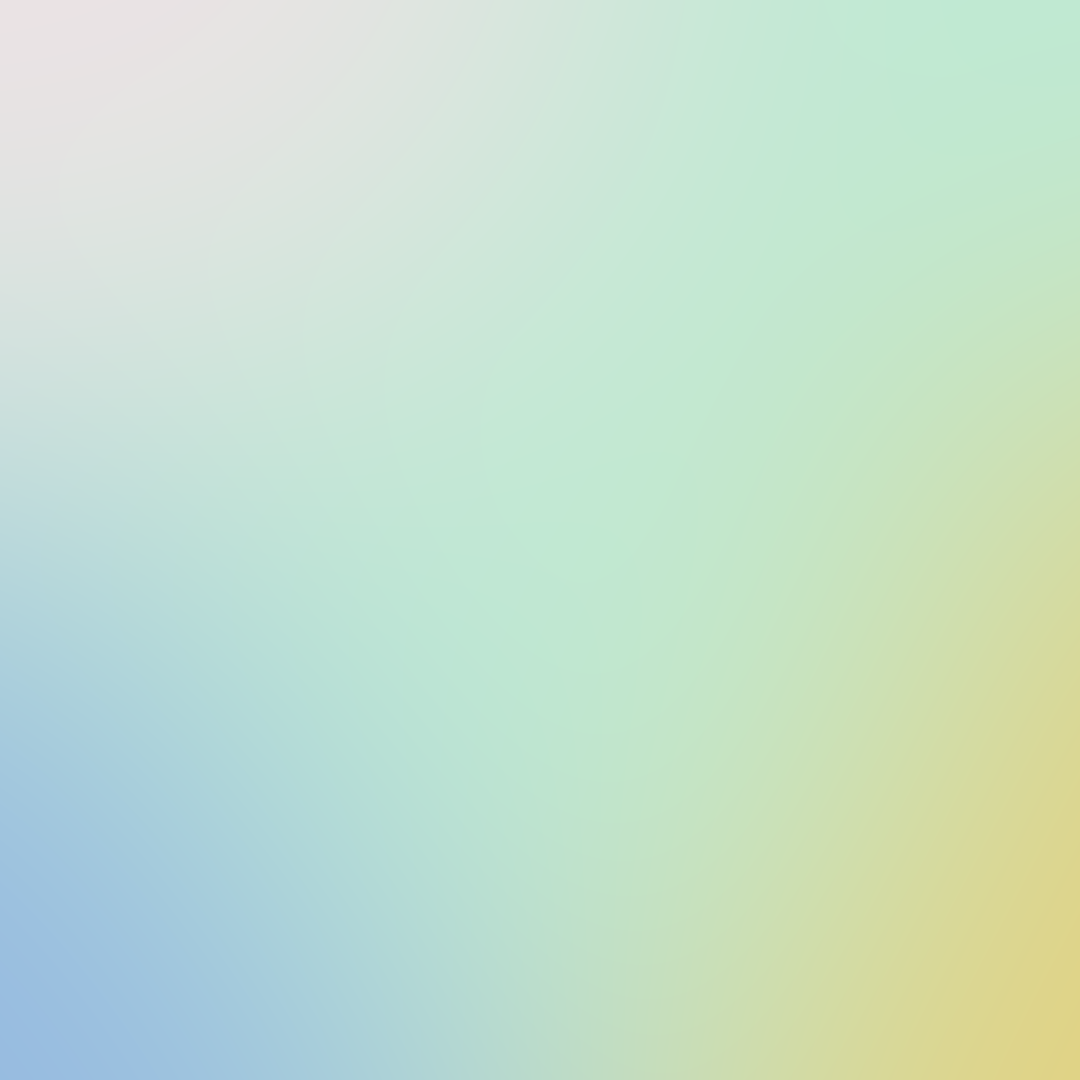
Attribution
Mentorship provided by Ben Kiel
Inspiration from Andy Clymer and Agyei Archer
Feedback provided by the SQSH Steering Team